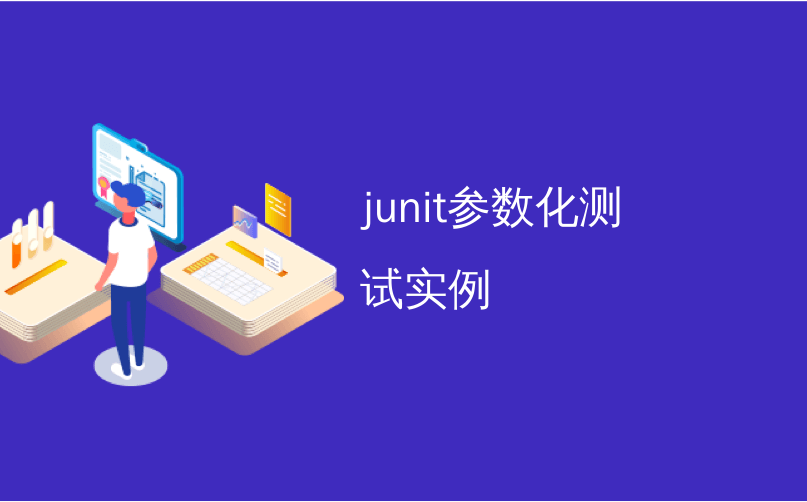
junit参数化测试实例
参数化测试允许您针对一组不同的数据运行测试。 如果您发现自己一次又一次地调用相同的测试但具有不同的输入,则参数化测试将有助于使代码更清晰。 要在JUnit 5中创建一个,您需要:
- 使用
@ParameterizedTest
注释测试方法 - 用至少一个来源注释测试方法,例如
@ValueSource
- 在测试方法中使用参数
以下各节描述了一些常用的源注释,您可以使用它们为测试方法提供输入。
@ValueSource此注释使您可以指定一个文字值数组,该数组将一一传递给测试方法,如以下示例所示:
@ParameterizedTest@ValueSource (ints = { 2 , 4 , 6 })void testIsEven( final int i) {assertTrue(i % 2 == 0 );}
@CsvSource此批注允许您指定一个逗号分隔值的数组,如果您的测试方法带有多个参数,则此注释很有用。 如果您有大量参数,则可以使用ArgumentsAccessor
提取参数,而不用创建带有长参数列表的方法。 例如:
@ParameterizedTest (name = "Person with name {0} and age {1}" )@CsvSource ({ "Alice, 28" ,"Bob, 30" })void testPerson( final String name, final int age) {final Person p = new Person(name, age);assertThat(p.getName(), is(name));assertThat(p.getAge(), is(age));}@ParameterizedTest (name = "Person with name {0} and age {1}" )@CsvSource ({ "Alice, 28" ,"Bob, 30" })void testPersonWithArgumentAccessor( final ArgumentsAccessor arguments) {final String name = arguments.getString( 0 );final int age = arguments.getInteger( 1 );final Person p = new Person(name, age);assertThat(p.getName(), is(name));assertThat(p.getAge(), is(age));}
顺便说一句,请注意如何使用{0}
和{1}
参数占位符自定义测试的显示名称。
@CsvFileSource该注释与CsvSource
相似,但是允许您从类路径上的CSV文件加载测试输入。 例如:
@ParameterizedTest (name = "Person with name {0} and age {1}" )@CsvFileSource (resources = { "data.csv" })void testPerson( final String name, final int age) {final Person p = new Person(name, age);assertThat(p.getName(), is(name));assertThat(p.getAge(), is(age));}
@MethodSource该批注允许您指定一种工厂方法,该方法返回要传递给测试方法的对象流。 如果您的测试方法有多个参数,则您的工厂方法应返回一个Arguments
实例流,如下例所示:
import static org.junit.jupiter.params.provider.Arguments.*;@ParameterizedTest (name = "{0} is sorted to {1}" )@MethodSource ( "dataProvider" )void testSort( final int [] input, final int [] expected) {Arrays.sort(input);assertArrayEquals(expected, input);}static Stream<Arguments> dataProvider() {return Stream.of(arguments( new int [] { 1 , 2 , 3 }, new int [] { 1 , 2 , 3 }),arguments( new int [] { 3 , 2 , 1 }, new int [] { 1 , 2 , 3 }),arguments( new int [] { 5 , 5 , 5 }, new int [] { 5 , 5 , 5 }));}
有关更多信息,请参见《 JUnit 5用户指南关于参数化测试》 。
如果您仍在使用JUnit 4(为什么?!),请查看我以前关于JUnit 4中的参数化测试的文章。
翻译自: https://www.javacodegeeks.com/2020/12/parameterized-tests-in-junit-5.html
junit参数化测试实例